Protect Your Business Access — Starting With API Keys
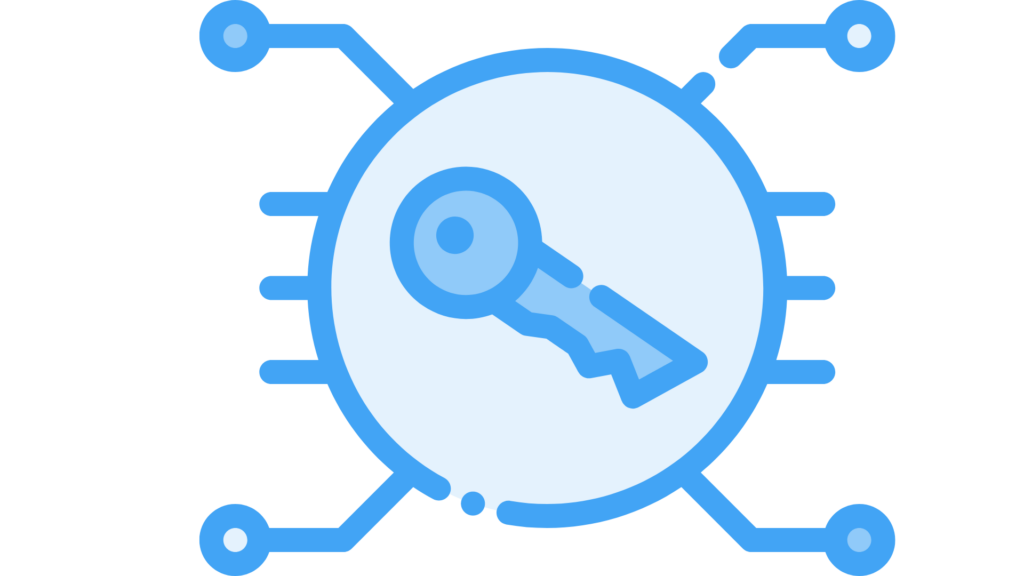
Let’s talk about something that’s often ignored but absolutely essential: your integration credentials.
Securing API keys is crucial because they act as unique identifiers and secret tokens, controlling access to sensitive data and functionality, and their compromise can lead to unauthorized access, data breaches, and system disruptions.
Why Secure Access Credentials Matter
If someone gets access to the API connections for Ticket Evolution, TicketNetwork, VividSeats, or any major exchange, they can:
- Download every order you’ve ever made
- Access your full customer base
- View and manipulate your ticket inventory
- And more
With just a few lines of code, your entire operation could be exposed.
That’s why who you trust with your credentials matters — a lot
You Control the Hosting and Platform
We build your WordPress-based ticketing site and let you host it anywhere — AWS, GoDaddy, Liquid Web, whatever you want.
You get ownership and freedom.
Control Your Own Integration Setup
With ticketWP, if you want we can install the site, then you change the admin password before you ever paste in your credentials. That way, only you have access.
We never store your access keys — and we never need to.
Built-In Website Security and Developer Access
At ticketWP, protecting your platform is built into our DNA. You get full developer access:
- FTP and SSH
- WordPress theme and template code
- Drag-and-drop page builders
- Plugin flexibility and control
and more!
If You’re Hosting With a Provider Who Sells Tickets…
Let’s be real — if your current provider also sells tickets, they’re your competitor.
And if they also have your connection credentials… that’s a problem. The ticket exchange is different — they already have all your information.
You’re not just sharing hosting — you’re sharing your business.
Real Example: Test It for Yourself
We built a simple PHP script — 283 lines — that connects to the Ticket Evolution API and shows all your orders. It even lets you export everything as a CSV.
If you’re unsure, copy the script into ChatGPT, Claude, or Grok and ask:
“What does this code do? Is it safe?”
Then try it on your local machine or server with your real keys.
You’ll see how powerful that access is. Now imagine if I built a system that matched your inventory against mine, downloaded your customers in real time, and tracked what tickets you purchased — when, how much — the sky’s the limit!
We’re building one for TicketNetwork next — just to show how exposed your platform might be.
ticketWP Gives You Ownership, Freedom, and Peace of Mind
Beyond pricing, beyond support, beyond design flexibility — this is about security.
If your API credentials are in the hands of someone who also competes with you, it’s time to move.
We’re here to educate, support, and give you full control of your digital foundation.
Learn more about Ticket Brokers
Best practices for securely using API keys
<?php
$TE_API_TOKEN_VALUE = 'ENTER_YOUR_TOKEN_HERE';
$TE_API_SECRET_VALUE = 'ENTER_YOUR_SECRET_HERE';
$TE_API_OFFICE_ID_VALUE = 'ENTER_YOUR_OFFICE_ID_HERE';
define('TE_API_URL', 'https://api.ticketevolution.com');
define('TE_API_TOKEN', $TE_API_TOKEN_VALUE);
define('TE_API_SECRET', $TE_API_SECRET_VALUE);
define('TE_API_OFFICE_ID', $TE_API_OFFICE_ID_VALUE);
// Get and process date parameters
$startDate = !empty($_GET['start_date']) ? $_GET['start_date'] : date("Y-m-d", strtotime("-30 days"));
$endDate = !empty($_GET['end_date']) ? $_GET['end_date'] : date("Y-m-d");
// Pagination Setup
$perPage = 20;
$page = isset($_GET['page']) ? (int)$_GET['page'] : 1;
// Build API query parameters
$queryParams = [
"office_id" => TE_API_OFFICE_ID,
"page" => $page,
"per_page" => $perPage
];
// Only add date filters if dates are set and not the default range
if (!empty($_GET['start_date']) || !empty($_GET['end_date'])) {
$queryParams["updated_at.gte"] = $startDate;
$queryParams["updated_at.lte"] = $endDate;
}
// Add other filter parameters
$allowedFilters = ['q', 'seller_id', 'buyer_id', 'order_id', 'state', 'type', 'event_date'];
foreach ($allowedFilters as $filter) {
if (!empty($_GET[$filter])) {
$queryParams[$filter] = ($filter === 'seller_id' || $filter === 'buyer_id' || $filter === 'order_id') ? (int)$_GET[$filter] : $_GET[$filter];
}
}
// Handle CSV export
if (isset($_GET['export']) && $_GET['export'] === 'csv') {
header('Content-Type: text/csv');
header('Content-Disposition: attachment;filename="orders_filtered.csv"');
$output = fopen('php://output', 'w');
fputcsv($output, ['Order Received', 'Order ID', 'Buyer', 'Seller', 'Event', 'Tickets', 'Total Amount', 'State']);
// Use the same query parameters but with more results per page
$exportParams = $queryParams;
$exportParams['per_page'] = 100;
$queryString = http_build_query($exportParams);
$method = "GET";
$host = "api.ticketevolution.com";
$path = "/v9/orders";
// Generate signature - using the method from the successful test
$string_to_sign = "$method $host$path?$queryString";
$signature = base64_encode(hash_hmac('sha256', $string_to_sign, TE_API_SECRET, true));
$url = "https://$host$path?$queryString";
$headers = [
"X-Token: " . TE_API_TOKEN,
"X-Signature: " . $signature,
"Accept: application/json"
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
$response = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
curl_close($ch);
if ($httpCode !== 200) {
die("Error: Unable to fetch orders for CSV. HTTP Code: $httpCode. Response: $response");
}
$data = json_decode($response, true);
if (!isset($data['orders']) || empty($data['orders'])) {
die("No orders found matching your criteria for CSV export.");
}
foreach ($data['orders'] as $order) {
$item = $order['items'][0] ?? null;
$event = $item && isset($item['ticket_group']['event']) ? $item['ticket_group']['event'] : null;
$eventInfo = $event ? $event['name'] . " " . date("Y-m-d H:i", strtotime($event['occurs_at'] ?? 'now')) : 'N/A';
$ticketInfo = $item ? "Qty: " . ($item['quantity'] ?? 'N/A') . " Price: $" . number_format($item['price'] ?? 0, 2) : 'N/A';
$buyerInfo = ($order['buyer']['name'] ?? 'N/A') . " (ID: " . ($order['buyer']['id'] ?? 'N/A') . ") Brokerage: " . ($order['buyer']['brokerage']['name'] ?? 'N/A');
$sellerInfo = ($order['seller']['name'] ?? 'N/A') . " (ID: " . ($order['seller']['id'] ?? 'N/A') . ") Brokerage: " . ($order['seller']['brokerage']['name'] ?? 'N/A');
fputcsv($output, [
date("Y-m-d H:i:s", strtotime($order['created_at'] ?? 'now')),
$order['id'] ?? 'N/A',
$buyerInfo,
$sellerInfo,
$eventInfo,
$ticketInfo,
"$" . number_format($order['total'] ?? 0, 2),
$order['state'] ?? 'N/A'
]);
}
fclose($output);
exit;
}
// For main page display
$queryString = http_build_query($queryParams);
$method = "GET";
$host = "api.ticketevolution.com";
$path = "/v9/orders";
// Generate signature
$string_to_sign = "$method $host$path?$queryString";
$signature = base64_encode(hash_hmac('sha256', $string_to_sign, TE_API_SECRET, true));
$url = "https://$host$path?$queryString";
$headers = [
"X-Token: " . TE_API_TOKEN,
"X-Signature: " . $signature,
"Accept: application/json"
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
$response = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
curl_close($ch);
if ($httpCode !== 200) {
die("Error: Unable to fetch orders. HTTP Code: $httpCode. Response: $response");
}
$data = json_decode($response, true);
if (!isset($data['orders']) || empty($data['orders'])) {
die("No orders found matching your criteria.");
}
$totalEntries = $data['total_entries'] ?? 0;
$totalPages = ceil($totalEntries / $perPage);
function sortUrl($field, $currentSort, $currentOrder) {
$order = ($currentSort === $field && $currentOrder === 'asc') ? 'desc' : 'asc';
return '?' . http_build_query(array_merge($_GET, ['sort' => $field, 'order' => $order]));
}
?>
<!DOCTYPE html>
<html>
<head>
<title>Ticket Evolution Orders</title>
<style>
table { border-collapse: collapse; width: 100%; }
th, td { border: 1px solid #ddd; padding: 8px; text-align: left; }
th { background-color: #f2f2f2; cursor: pointer; }
th a { text-decoration: none; color: black; }
.filter-form { margin-bottom: 20px; }
.pagination { margin-top: 20px; }
.export-btn { margin-bottom: 20px; }
</style>
</head>
<body>
<h2>Ticket Evolution Orders</h2>
<div class="filter-form">
<form method="GET">
<label>Start Date: <input type="date" name="start_date" value="<?php echo htmlspecialchars($startDate); ?>"></label>
<label>End Date: <input type="date" name="end_date" value="<?php echo htmlspecialchars($endDate); ?>"></label>
<label>Search: <input type="text" name="q" value="<?php echo htmlspecialchars($_GET['q'] ?? ''); ?>" placeholder="Brokerage/Client name or ID"></label>
<label>Seller ID: <input type="number" name="seller_id" value="<?php echo htmlspecialchars($_GET['seller_id'] ?? ''); ?>"></label>
<label>Buyer ID: <input type="number" name="buyer_id" value="<?php echo htmlspecialchars($_GET['buyer_id'] ?? ''); ?>"></label>
<label>Order ID: <input type="number" name="order_id" value="<?php echo htmlspecialchars($_GET['order_id'] ?? ''); ?>"></label>
<label>State:
<select name="state">
<option value="">All</option>
<option value="pending" <?php echo ($_GET['state'] ?? '') === 'pending' ? 'selected' : ''; ?>>Pending</option>
<option value="accepted" <?php echo ($_GET['state'] ?? '') === 'accepted' ? 'selected' : ''; ?>>Accepted</option>
<option value="rejected" <?php echo ($_GET['state'] ?? '') === 'rejected' ? 'selected' : ''; ?>>Rejected</option>
<option value="completed" <?php echo ($_GET['state'] ?? '') === 'completed' ? 'selected' : ''; ?>>Completed</option>
</select>
</label>
<label>Type:
<select name="type">
<option value="">All</option>
<option value="Order" <?php echo ($_GET['type'] ?? '') === 'Order' ? 'selected' : ''; ?>>Sale</option>
<option value="PurchaseOrder" <?php echo ($_GET['type'] ?? '') === 'PurchaseOrder' ? 'selected' : ''; ?>>Purchase</option>
</select>
</label>
<input type="submit" value="Filter">
</form>
</div>
<div class="export-btn">
<a href="?<?php echo http_build_query(array_merge($_GET, ['export' => 'csv'])); ?>">Export to CSV (Filtered)</a>
</div>
<table>
<tr>
<th><a href="<?php echo sortUrl('created_at', $sortField ?? '', $sortOrder ?? ''); ?>">Order Received</a></th>
<th><a href="<?php echo sortUrl('id', $sortField ?? '', $sortOrder ?? ''); ?>">Order ID</a></th>
<th>Buyer</th>
<th>Seller</th>
<th>Event</th>
<th>Tickets</th>
<th><a href="<?php echo sortUrl('total', $sortField ?? '', $sortOrder ?? ''); ?>">Total Amount</a></th>
<th><a href="<?php echo sortUrl('state', $sortField ?? '', $sortOrder ?? ''); ?>">State</a></th>
</tr>
<?php foreach ($data['orders'] as $order): ?>
<tr>
<td><?php echo isset($order['created_at']) ? date("Y-m-d H:i:s", strtotime($order['created_at'])) : 'N/A'; ?></td>
<td><?php echo htmlspecialchars($order['id'] ?? 'N/A'); ?></td>
<td>
<?php
if (isset($order['buyer'])) {
$buyer = $order['buyer'];
echo htmlspecialchars($buyer['name'] ?? 'N/A') . " (ID: " . htmlspecialchars($buyer['id'] ?? 'N/A') . ")<br>";
echo "Brokerage: " . htmlspecialchars($buyer['brokerage']['name'] ?? 'N/A');
} else {
echo 'N/A';
}
?>
</td>
<td>
<?php
if (isset($order['seller'])) {
$seller = $order['seller'];
echo htmlspecialchars($seller['name'] ?? 'N/A') . " (ID: " . htmlspecialchars($seller['id'] ?? 'N/A') . ")<br>";
echo "Brokerage: " . htmlspecialchars($seller['brokerage']['name'] ?? 'N/A');
} else {
echo 'N/A';
}
?>
</td>
<td>
<?php
$item = isset($order['items'][0]) ? $order['items'][0] : null;
$event = $item && isset($item['ticket_group']['event']) ? $item['ticket_group']['event'] : null;
if ($event) {
echo htmlspecialchars($event['name'] ?? 'N/A') . "<br>";
echo date("Y-m-d H:i", strtotime($event['occurs_at'] ?? 'now'));
} else {
echo "N/A";
}
?>
</td>
<td>
<?php
if ($item) {
echo "Qty: " . htmlspecialchars($item['quantity'] ?? 'N/A') . "<br>";
echo "Price: $" . number_format($item['price'] ?? 0, 2);
} else {
echo "N/A";
}
?>
</td>
<td>$<?php echo number_format($order['total'] ?? 0, 2); ?></td>
<td><?php echo htmlspecialchars($order['state'] ?? 'N/A'); ?></td>
</tr>
<?php endforeach; ?>
</table>
<div class="pagination">
<?php if ($page > 1): ?>
<a href="?<?php echo http_build_query(array_merge($_GET, ['page' => $page - 1])); ?>">Previous Page</a> |
<?php endif; ?>
<span>Page <?php echo $page; ?> of <?php echo $totalPages; ?></span>
<?php if ($page < $totalPages): ?>
| <a href="?<?php echo http_build_query(array_merge($_GET, ['page' => $page + 1])); ?>">Next Page</a>
<?php endif; ?>
</div>
</body>
</html>